Today, I am listing some of the basic concepts in Operating Systems.
* Quick warning - this may be very simple.
- Program v. Process v. Thread
- Program: executable file that includes process instructions or code.
- When the program is loaded from the disk to the main memory (RAM) and executed by a processor, it becomes a process.
- Process: an instance of execution - running code.
- Includes resources like program registers, program counter(PC), page management, stack, heap, etc.
- Each processor has its own memory space -> safe but expensive.
- "Traditional process" refers to the single-core process with one thread.
- Thread: the smallest unit of execution - a unit of CPU utilization within the same address space.
- Has own stack, register, and PC; shares heap space in the process.
- One process can have multiple threads (user threads).
- Multiple share the address space -> fast communication but blocking issue.
- Program: executable file that includes process instructions or code.
- User-threads v. Kernel-threads
- User-level thread: task created and managed in user space (kernel can't see).
- pro: excellent performance in parallel programming, low-cost, flexible
- con: no I/O operations (blocking call blocks the whole threads), poor perf when built on top of traditional processes.
- Kernel thread: task created & managed by the kernel/OS.
- pro: no system integration problems like user-level threads
- con: too heavyweight for efficient parallel programming.
- Does the Linux schedule process or thread?
- it schedules a single-threaded process (fork), user-level thread (thread in multi-threaded process), and kernel task (kernel thread).
- User-level thread: task created and managed in user space (kernel can't see).
- Stack, Heap, Code, Data in Process
- Stack: stores local variables, function parameters, return address
- Last-In-First-Out
- When the function is called, its local variables & parameters are allocated on the stack. As it exits, the function's allocated space is deallocated (auto).
- grows @ function call, shrinks @ function return. --> dynamic size
- stack overflow: when excessive allocation/deep function call chain happens with limited stack memory.
- Heap: dynamic memory allocation
- allocated with malloc, calloc, realloc; deallocated with free (not auto). --> dynamic size
- to handle data structures with dynamic sizes like array (size determined at runtime), and linked lists.
- improper use can lead to memory leaks (no free) or fragmentation (mem holes).
- Data / Initialized Data: initialized global variables, initialized constant global variables, initialized local static variables.
- ex) int globalVar=1; or static int localStatic=1;
- consumes space in the executable file to store the variable with initial values.
- In summary, the data segment is utilized for storing initialized variables along with their values, and this allocation occurs within the executable file, contributing to the program's memory layout.
- BSS (Block Started by Symbol) / Uninitialized Data: uninitialized global variables, uninitialized constant global variables, uninitialized local static variables.
- ex) int globalVar; or static int localStatic;
- global variables declared as 0 or NULL also stored @ BSS.
- reduces the size of the executable.
- Code (Text): frequently executed/compiled machine code
- often read-only to avoid risks like buffer overflow/segmentation faults/program crash.
- Stack: stores local variables, function parameters, return address
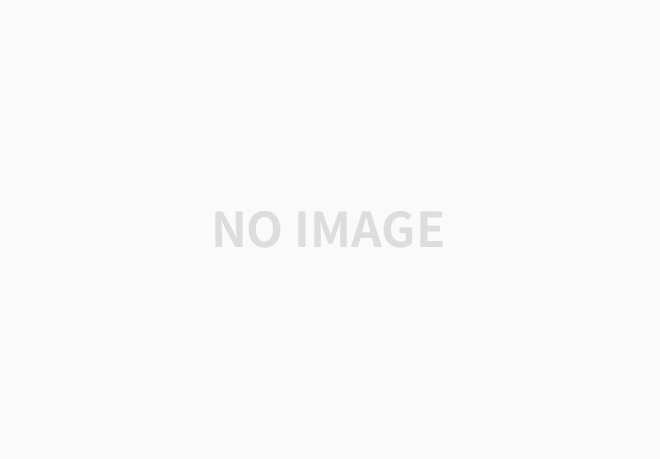
- Local v. Global v. Static variables
- local: only exists in the function where it's created & disappears when the function ends.
- in stack (declared & defined in functions)
- two local variables in different functions are completely independent of each other.
- local: only exists in the function where it's created & disappears when the function ends.
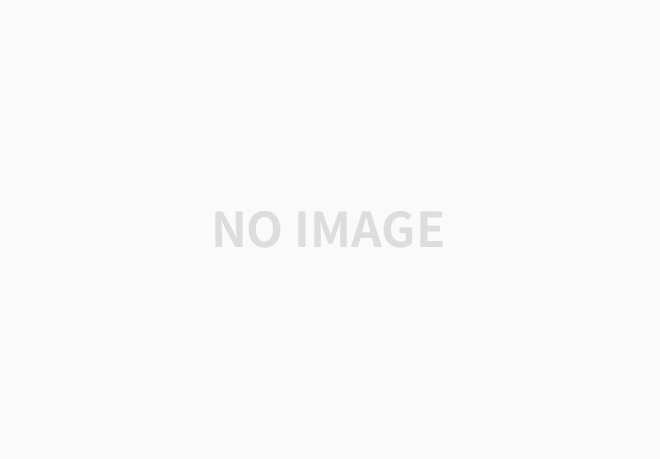
To change the local var outside of the function, use pointer
-
- global: available to all functions until the program ends. ----> data
- even can be accessed by another file with "extern".
- static: only accessible by the file where it's created. ----> data
- can be local (defined in the function)/global (outside of function) - default is global.
- local var: gets re-initialized when the function is called.
- local static var: still exists in the memory after the function ends, but can only access from that function.
- global: available to all functions until the program ends. ----> data
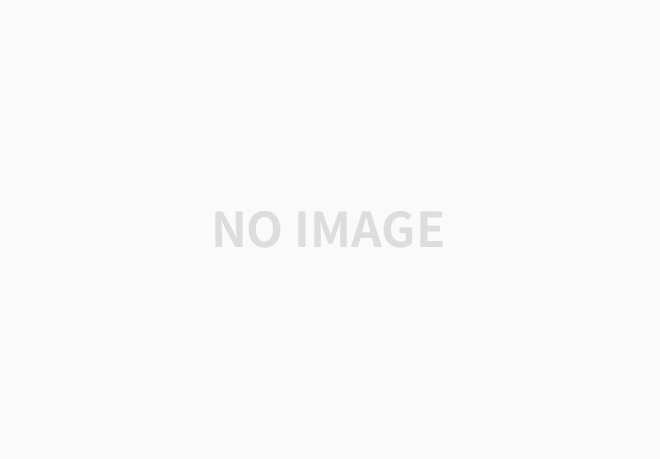
- constant: #define replace the variable with the appropriate value. only exists in the file where it's created. ----> code and/or data
- problem when the same definition is in another file with a different value.
- Volatile variable: tells the compiler not to optimize.
- C compilers optimize the code or skip the abstraction when converting to actual machine code like the following picture:
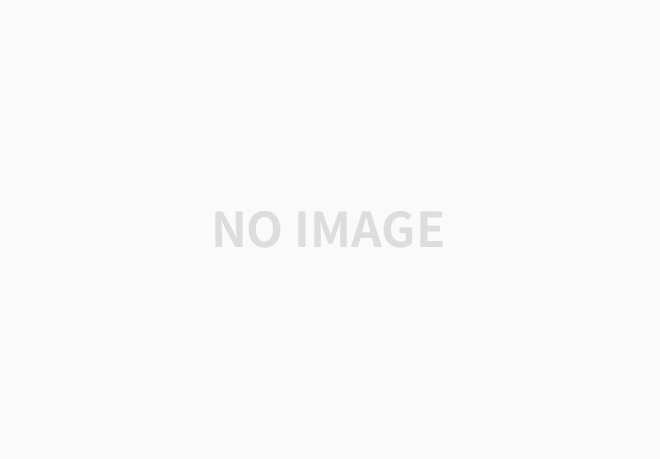
- C establish a connection between the abstract and actual machines:
- the arguments to main()
- the return value from main()
- the side-effecting functions in the C standard library
- volatile variables
- When to use:
- When you interface with hardware that changes the value itself (Memory-mapped peripheral registers)
- when there's another thread running that also uses the variable (Global variables within a multi-threaded application)
- when there's a signal handler that might change the value of the variable (Global variables modified by an interrupt service routine)
'OS' 카테고리의 다른 글
[OS] Lock, Semaphore, Monitor (0) | 2023.12.13 |
---|